Supporting classes
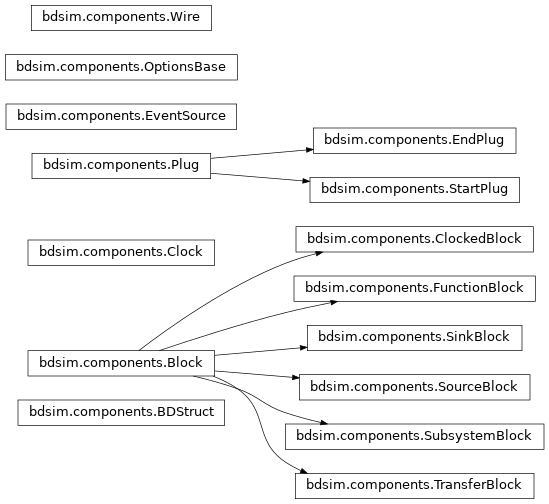
BDSim class
This class describes the run-time environment for executing a block diagram.
- class bdsim.BDSim(banner=True, packages=None, load=True, toolboxes=True, **kwargs)[source]
Bases:
object
- __init__(banner=True, packages=None, load=True, toolboxes=True, **kwargs)[source]
- Parameters:
banner (bool, optional) – display docstring banner, defaults to True
packages (str) – colon-separated list of folders to search for blocks
load (bool,optional) – dynamically load blocks from libraries, defaults to True
sysargs (bool, optional) – process options from sys.argv, defaults to True
graphics (bool, optional) – enable graphics, defaults to True
animation (bool, optional) – enable animation, defaults to False
progress (bool, optional) – enable progress bar, defaults to True
debug (str, optional) – debug options, defaults to None
backend (str, optional) – matplotlib backend, defaults to ‘Qt5Agg’’
tiles (str, optional) – figure tile layout on monitor, defaults to ‘3x4’
- Raises:
ImportError – syntax error in block
- Returns:
parent object for blockdiagram simulation
- Return type:
If
sysargs
is True, process command line arguments and passed options. Command line arguments have precedence.Command line switch
Argument
Default
Behaviour
–graphics, +g
graphics
True
enable graphical display
–animation, +a
animation
True
update graphics at each time step
–hold, +h
hold
True
hold graphics in done()
–no-graphics, -g
graphics
True
disable graphical display
–no-animation, -a
animation
True
don’t update graphics at each time step
–no-hold, -H
hold
True
do not hold graphics in done()
–no-progress, -p
progress
True
do not display simulation progress bar
–backend BE
backend
‘Qt5Agg’
matplotlib backend
–tiles RxC, -t RxC
tiles
‘3x4’
arrangement of figure tiles on the display
–shape WxH
shape
None
window size, default matplotlib size
–altscreen, +A,
altscreen
True
display plots on second monitor
–no-altscreen, -A
altscreen
True
do not display plots on second monitor
–debug F, -d F
debug
‘’
debug flag string
–simtime T[,dt]
simtime
(10,)
simulation time
–verbose, -v
verbose
False
be verbose
–quiet, -q
quiet
False
suppress reports
-o
outfile
None
output pickled simulation results to bd.out
–out OUTFILE
outfile
None
file to save pickled simulation results
–set P, -s P
setparam
[]
override block parameter using
P=block:param=value
–global G
setglob
[]
override global parameter using
G=var=value
Note
animation
andgraphics
options are coupled. Ifgraphics=False
, all graphics is suppressed. Ifgraphics=True
then graphics are shown and the behaviour depends onanimation
.animation=False
shows graphs at the end of the simulation, while ``animation=True` will animate the graphs during simulation.- Seealso:
- blockdiagram(name='main')[source]
Instantiate a new block diagram object.
- Parameters:
name (str, optional) – diagram name, defaults to ‘main’
- Returns:
parent object for blockdiagram
- Return type:
This object describes the connectivity of a set of blocks and wires.
It is an instantiation of the
BlockDiagram
class with a factory method for every dynamically loaded block which returns an instance of the block. These factory methods have names which are all upper case, for example, the method.GAIN
invokes the constructor for theGain
class.- Seealso:
- blockinfo(block=None)[source]
Return info about all blocks
- Parameters:
block (str, optional) – name of block to return info for, otherwise list of info for all
- Returns:
parameters of blocks
- Return type:
Detailed metadata about a block is obtained by introspection and parsing the block’s docstring.
Key
Description
path
Path to the folder containing block definition
classname
Name of class
url
URL of online documentation
class
Reference to the class
module
Name of the module package.blocks.module
package
Name of the package, eg. bdsim, roboticstoolbox
params
Dict of (type, descrip), indexed by parameter name
inputs
List of names of block inputs
outputs
List of names of block outputs
nin
Number of inputs, -1 if variable
nout
Number of outputs, -1 if variable
blockclass
Block class, eg. source, sink etc.
- blocks()[source]
List all loaded blocks.
Example:
73 blocks loaded bdsim.blocks.functions..................: Sum Prod Gain Clip Function Interpolate bdsim.blocks.sources....................: Constant Time WaveForm Piecewise Step Ramp bdsim.blocks.sinks......................: Print Stop Null Watch bdsim.blocks.transfers..................: Integrator PoseIntegrator LTI_SS LTI_SISO bdsim.blocks.discrete...................: ZOH DIntegrator DPoseIntegrator bdsim.blocks.linalg.....................: Inverse Transpose Norm Flatten Slice2 Slice1 Det Cond bdsim.blocks.displays...................: Scope ScopeXY ScopeXY1 bdsim.blocks.connections................: Item Dict Mux DeMux Index SubSystem InPort OutPort roboticstoolbox.blocks.arm..............: FKine IKine Jacobian Tr2Delta Delta2Tr Point2Tr TR2T FDyn IDyn Gravload ........................................: Inertia Inertia_X FDyn_X ArmPlot Traj JTraj LSPB CTraj CirclePath roboticstoolbox.blocks.mobile...........: Bicycle Unicycle DiffSteer VehiclePlot roboticstoolbox.blocks.uav..............: MultiRotor MultiRotorMixer MultiRotorPlot machinevisiontoolbox.blocks.camera......: Camera Visjac_p EstPose_p ImagePlane
- fatal(message, retval=1)[source]
Fatal simulation error
- Parameters:
Display the error message then terminate the process. For operating systems that support it, return an integer code.
- load_blocks(verbose=True, toolboxes=True)[source]
Dynamically load all block definitions.
- Raises:
ImportError – module could not be imported
- Returns:
dictionary of block metadata
- Return type:
Reads blocks from .py files found in bdsim/bdsim/blocks, folders given by colon separated list in envariable BDSIMPATH, and the command line option
packages
.The result is a dict indexed by the upper-case block name with elements: -
path
to the folder holding the Python file defining the block -classname
-blockname
, upper case version ofclassname
-url
of online documentation for the block -package
containing the block - doc is the docstring from the class constructor
- report(bd, type='summary', **kwargs)[source]
Print block diagram report
- Parameters:
bd (
BlockDiagram
) – the block diagram to be reportedtype (str, optional) – report type, one of: “summary” (default), “lists”, “schedule”
style (str) – table style, one of: ansi (default), markdown, latex
Single method wrapper for various block diagram reports. Obeys the
-q
option to suppress all reports at runtime.
- run(bd, T=5, dt=None, solver='RK45', solver_args={}, debug='', block=None, checkfinite=True, minstepsize=1e-12, watch=[])[source]
Run the block diagram
- Parameters:
T (float, optional) – maximum integration time, defaults to 10.0
dt (float, optional) – maximum time step
solver (str, optional) – integration method, defaults to
RK45
block (bool) – matplotlib block at end of run, default False
checkfinite (bool) – error if inf or nan on any wire, default True
minstepsize (float) – minimum step length, default 1e-6
watch (list) – list of input ports to log
solver_args (dict) – arguments passed to
scipy.integrate
- Returns:
time history of signals and states
- Return type:
Sim class
Assumes that the network has been compiled.
The system is simulated from time 0 to
T
.The integration step time
dt
defaults toT/100
but can be specified. Finer control can be achieved usingmax_step
andfirst_step
parameters to the underlying integrator using thesolver_args
parameter.Results are returned in a class with attributes:
t
the time vector: ndarray, shape=(M,)x
is the state vector: ndarray, shape=(M,N)xnames
is a list of the names of the states corresponding to columns of x, eg. “plant.x0”,defined for the block using the
snames
argument
yN
for a watched input where N is the index of the port mentioned in thewatch
argumentynames
is a list of the names of the input ports being watched, same order as inwatch
argument
If there are no dynamic elements in the diagram, ie. no states, then
x
andxnames
are not present.The
watch
argument is a list of one or more input ports whose value during simulation will be recorded. The elements of the list can be:a
Block
reference, which is interpretted as input port 0a
Plug
reference, ie. a block with an index or attributea string of the form “block[i]” which is port i of the block named block.
The debug string comprises single letter flags:
‘p’ debug network value propagation
‘s’ debug state vector
‘d’ debug state derivative
Note
Simulation stops if the step time falls below
minsteplength
which typically indicates that the solver is struggling with a very harsh non-linearity.
- run_interval(bd, t0, T, x0, simstate=None)[source]
Integrate system over interval
- Parameters:
bd (BlockDiagram) – the system blockdiagram
t0 (float) – initial time
tf (float) – final time
x0 (ndarray(n)) – initial state vector
simstate (SimState) – simulation state object
- Returns:
final state vector xf
- Return type:
ndarray(n)
The system is integrated from from
x0
toxf
over the intervalt0
totf
.
- set_globals(globs)[source]
Set globals as specified by command line
- Parameters:
globs (dict) – global variables
The command line option
--global var=value
can be used to request the change of global variables. However, actually changing them requires explicit code support in the user’s program after theBDSim
constructor.Example:
sim.set_globals(globals())
Messages are displayed by defaulting, indicating which variables are changed, and their old and new values.
- update_parameters(bd)[source]
Set value of parameters according to command line arguments
Command line arguments of the form:
-s block:param=value
--set block:param=value
are stored as list items in
options.setparam
block
can be either:the block’s name as a string, either user assigned or bdsim assigned
the block
id
as displayed by thereport
method
param
is the name of the parameter used in the constructorvalue
is the new value of the variable
- class bdsim.BDSimState[source]
Bases:
object
- Variables:
x (np.ndarray) – state vector
T (float) – maximum simulation time (seconds)
t (float) – current simulation time (seconds)
fignum (int) – number of next matplotlib figure to create
stop (Block subclass) – reference to block wanting to stop simulation, else None
checkfinite (bool) – halt simulation if any wire has inf or nan
graphics (bool) – enable graphics
BlockDiagram class
This class describes a block diagram, a collection of blocks and wires that can be “executed”.
- class bdsim.BlockDiagram(name='main', **kwargs)[source]
Bases:
object
Block diagram class. This object is the parent of all blocks and wires in the system.
- Variables:
wirelist (list of Wire instances) – all wires in the diagram
blocklist (list of Block subclass instances) – all blocks in the diagram
x (np.ndarray) – state vector
compiled (bool) – diagram has successfully compiled
blockcounter (collections.Counter) – unique counter for each block type
blockdict (dict of lists) – index of all blocks by category
name (str) – name of this diagram
This object:
holds all the blocks and wires that comprise the system
manages continuous- and discrete-time state vector for the whole system, splitting it across blocks as required
evaluates the entire diagram as a function to compute
dot{x} = f(x, t)()
- compile(subsystem=False, doimport=True, evaluate=True, report=False, verbose=True)[source]
Compile the block diagram
- Parameters:
- Raises:
RuntimeError – various block diagram errors
- Returns:
Compile status
- Return type:
Performs a number of operations:
Check sanity of block parameters
Recursively clone and import subsystems
Check for loops without dynamics
Check for inputs driven by more than one wire
Check for unconnected inputs and outputs
Link all output ports to outgoing wires
Link all input ports to incoming wires
Evaluate all blocks in the network
- connect(start, *ends, name=None)[source]
- TODO:
s.connect(out[3], in1[2], in2[3]) # one to many block[1] = SigGen() # use setitem block[1] = SumJunction(block2[3], block3[4]) * Gain(value=2)
- deriv(t)[source]
Harvest derivatives from all blocks.
- Parameters:
t (float) – simulation time, defaults to None
simstate (SimState, optional) – simulation state, defaults to None
- done(block=False)[source]
Finishup all blocks
- Parameters:
state (SimState, optional) – simulation state, defaults to None
graphics (bool, optional) – graphics enabled, defaults to False
Inform all blocks that BlockDiagram execution is complete by invoking their
done
method and passing options. Used to close files, display figures etc.Note
if
graphics
is False, Graphics blocks are not called
- dotfile(filename, shapes=None)[source]
Write a GraphViz dot file representing the network.
- Parameters:
Create a GraphViz format file for procesing by
dot
. The graph is:directed graph, drawn left to right
source blocks are in the first column
sink and graphics blocks are in the last column
SUM
andPROD
blocks have the sign or operation of their input wires labeled.
The file can be processed using
dot
:% dot -Tpng -o out.png dotfile.dot
Note
By default all blocks have the default shape, with source blocks shown as a rectangle (“record”), and sink/graphics blocks as a rounded rectangle (“Mrecord”). This can be overriden by provide a dictionary
shapes
that maps block class (sink, source, graphics, function, transfer) to the names of GraphViz shapes.- Seealso:
- property issubsystem
- report_lists(**kwargs)[source]
Print a tabular report about the block diagram.
- Parameters:
kwargs – options passed to
ansitable.ANSITable.print()
Print the important lists in pretty format.
block list, all blocks
wire list, all wires
clock list, all discrete time clocks
- report_schedule(**kwargs)[source]
Display execution schedule in tabular form
- Parameters:
kwargs – options passed to
ansitable.ANSITable.print()
- Seealso:
schedule_plan()
,schedule_dotfile()
- report_summary(sortby='name', **kwargs)[source]
Print a summary of block diagram.
- Parameters:
Print a table with 4 columns:
Block name, sorted in alphabetical order
The input port (if not a source block)
The block driving this port (if not a source block)
The type of value driving this port (if not a source block)
If the block is an event source, add a
@
suffix.
- reset()[source]
Reset conditions within every active block. Most importantly, all inputs are marked as unknown.
Invokes the reset method on all blocks.
- schedule_dotfile(filename)[source]
Write a GraphViz dot file representing the execution schedule
- Parameters:
file (str) – Name of file to write to
The file can be processed using neato or dot:
% dot -Tpng -o out.png dotfile.dot
Display execution plan as a dataflow graph.
- Seealso:
schedule_plan()
,schedule_print()
- schedule_evaluate(x, t, checkfinite=True, sinks=True, simstate=None)[source]
Evaluate all blocks in the network
- Parameters:
- Returns:
state derivative
- Return type:
Performs the following steps:
Partition the state vector
x
to all stateful blocksExecute the blocks in the order given by the
plan
. The block outputs are “sent” to their connected inputs.
Sink blocks are not executed here, but after completion their inputs will all be valid.
- schedule_generate()[source]
Create execution plan
The plan is saved in the attribute
plan
and is a list[L0, L1, ... LN]
where eachLi
is a list of blocks. The blocks in the lists are executed sequentially, ie. all the blocks inL0
then all the blocks inL1
etc.The plan ensures that the inputs of all blocks in
Li
have been previously computed.Note
The plan is essentially a dataflow graph.
The blocks in list
Li
could potentially be executed in parallel.Constant blocks and stateful blocks are all executed in
L0
The block attribute
_sequence
isi
and indicates its execution order
- Seealso:
schedule_report()
,schedule_dotfile()
- start(simstate=None)[source]
Start all blocks
- Parameters:
simstate (SimState, optional) – simulation state, defaults to None
Inform all blocks that BlockDiagram execution is about to start by invoking their
start
method and passing thestate
object. Used to open files, create figures etc.Note
if
graphics
is False, Graphics blocks are not called
Components
Wire
- class bdsim.Wire(start=None, end=None, name=None)[source]
Bases:
object
Create a wire.
- Parameters:
- Returns:
A wire object
- Return type:
A Wire object connects two block ports. A Wire has a reference to the start and end ports.
A wire records all the connections defined by the user. At compile time wires are used to build inter-block references.
Between two blocks, a wire can connect one or more ports, ie. it can connect a set of output ports on one block to a same sized set of input ports on another block.
- property fullname
Display wire connection details.
- Returns:
Wire name
- Return type:
String format:
d2goal[0] --> Kv[0]
- property info
Interactive display of wire properties.
Displays all attributes of the wire for debugging purposes.
Plug
- class bdsim.Plug(block, port=0, type=None)[source]
Bases:
object
Create a plug.
- Parameters:
- Returns:
Plug object
- Return type:
Plugs are the interface between a wire and block and have information about port number and wire end. Plugs are on the end of each wire, and connect a Wire to a specific port on a Block.
- The
type
argument indicates if thePlug
is at: the start of a wire, ie. the port is an output port
the end of a wire, ie. the port is an input port
A plug can specify a set of ports on a block.
- __repr__()[source]
Display plug details.
- Returns:
Plug description
- Return type:
String format:
bicycle.0[1]
- __str__()[source]
Display plug details.
- Returns:
Plug description
- Return type:
String format:
bicycle.0[1]
- property isslice
Test if port number is a slice.
- Returns:
Whether the port is a slice
- Return type:
Returns
True
if the port is a slice, eg.[0:3]
, andFalse
for a simple index, eg.[2]
.
Blocks
- class bdsim.Block(*args, bd=None, **kwargs)[source]
Bases:
object
- T_deriv(*inputs, t=0.0, x=None)[source]
Evaluate a block for unit testing.
- Parameters:
- Returns:
Block derivative value
- Return type:
ndarray
The derivative of the block is evaluated for a given set of input port values. Input port values are treated as lists.
Mostly used for making concise unit tests.
Warning
the instance is monkey patched, not useable in a block diagram subsequently.
- T_next(*inputs, t=0.0, x=None)[source]
Evaluate a block for unit testing.
- Parameters:
- Returns:
Block next state value
- Return type:
ndarray
The next value of a discrete time block is evaluated for a given set of input port values. Input port values are treated as lists.
Mostly used for making concise unit tests.
- T_output(*inputs, t=0.0, x=None)[source]
Evaluate a block for unit testing.
- Parameters:
*inputs –
Input port values
t (float, optional) – Simulation time, defaults to 0.0
x (ndarray) – state vector
- Returns:
Block output port values
- Return type:
The output ports of the block are evaluated for a given simulation time and set of input port values. Input ports are assigned to consecutive inputs, output port values are a list.
Mostly used for making concise unit tests.
Warning
the instance is monkey patched, not useable in a block diagram subsequently.
- T_step(*inputs, t=0.0)[source]
Step a block for unit testing.
- Parameters:
Step the block for a given set of input port values. Input port values are treated as lists.
Mostly used for making concise unit tests.
- __annotations__ = {}
- __getitem__(port)[source]
Convert a block slice reference to a plug.
Invoked whenever a block is referenced as a slice, for example:
c = bd.CONSTANT(1) bd.connect(x, c[0]) bd.connect(c[0], x)
In both cases
c[0]
is converted to aPlug
by this method.
- __init__(name=None, nin=None, nout=None, inputs=None, type=None, inames=None, onames=None, snames=None, pos=None, bd=None, blockclass=None, verbose=False, **kwargs)[source]
Construct a new block object.
- Parameters:
name (str, optional) – Name of the block, defaults to None
nin (int, optional) – Number of inputs, defaults to None
nout (int, optional) – Number of outputs, defaults to None
inputs (Block, Plug or list of Block or Plug) – Optional incoming connections
inames (list of str, optional) – Names of input ports, defaults to None
onames (list of str, optional) – Names of output ports, defaults to None
snames (list of str, optional) – Names of states, defaults to None
pos (2-element tuple or list, optional) – Position of block on the canvas, defaults to None
bd (BlockDiagram, optional) – Parent block diagram, defaults to None
verbose (bool, optional) – enable diagnostic prints, defaults to False
kwargs (dict) – Unused arguments
- Returns:
A Block superclass
- Return type:
A block object is the superclass of all blocks in the simulation environment.
This is the top-level initializer, and handles most options passed to the superclass initializer for each block in the library.
- static __new__(cls, *args, bd=None, **kwargs)[source]
Construct a new Block object.
- Parameters:
cls (class type) – The class to construct
*args –
positional args passed to constructor
**kwargs –
keyword args passed to constructor
- Returns:
new Block instance
- Return type:
Block instance
- __setattr__(name, value)[source]
Convert a LHS block name reference to a wire.
Used to create a wired connection by assignment, for example:
c = bd.CONSTANT(1, inames=['u']) c.u = x
Ths method is invoked to create a wire from
x
to port ‘u’ of the constant blockc
.Notes:
this overloaded method handles all instances of
setattr
and implements normal functionality as well, only creating a wire ifname
is a known port name.
- __setitem__(port, src)[source]
Convert a LHS block slice reference to a wire.
Used to create a wired connection by assignment, for example:
X[0] = Y
where
X
andY
are blocks. This method is implicitly invoked and creates a wire fromY
to input port 0 ofX
.Note
The square brackets on the left-hand-side is critical, and
X = Y
will simply overwrite the reference toX
.
- property info
Interactive display of block properties.
Displays all attributes of the block for debugging purposes.
- inport_names(names)[source]
Set the names of block input ports.
Invoked by the
inames
argument to the Block constructor.The names can include LaTeX math markup. The LaTeX version is used where appropriate, but the port names are a de-LaTeXd version of the given string with backslash, caret, braces and dollar signs removed.
- property inputs
Get block inputs as a list
- Returns:
list of block inputs
- Return type:
Returns a list of values corresponding to the input ports of the block. The types of the elements are dictated by the blocks connected to the input ports.
Note
When a block’s
output
method is evaluated the resulting list is saved as an attribute of that block. Theinputs
method uses thesources
attribute which has references to the output values held by the predecessor block.- Seealso:
input()
- property isclocked
Test if block is clocked
- Returns:
True if block is clocked
- Return type:
True if block is clocked, False if it is continuous time.
- property isgraphics
Test if block does graphics
- Returns:
True if block does graphics
- Return type:
- outport_names(names)[source]
Set the names of block output ports.
Invoked by the
onames
argument to the Block constructor.The names can include LaTeX math markup. The LaTeX version is used where appropriate, but the port names are a de-LaTeXd version of the given string with backslash, caret, braces and dollar signs removed.
- sourcename(port)[source]
Get the name of output port driving this input port.
Return the name of the output port that drives the specified input port. The name can be:
a LaTeX string if provided
block name with port number given in square brackets. The block name will the one optionally assigned by the user using the
name
keyword, otherwise a systematic default name.
- Seealso:
outport_names
- varinputs = False
- varoutputs = False
Source block
- class bdsim.SourceBlock(*args, bd=None, **kwargs)[source]
Bases:
Block
A SourceBlock is a subclass of Block that represents a block that has outputs but no inputs. Its output is a function of parameters and time.
- __annotations__ = {}
- __init__(**blockargs)[source]
Create a source block.
- Parameters:
blockargs (dict) – common Block options
- Returns:
source block base class
- Return type:
This is the parent class of all source blocks.
- __module__ = 'bdsim.components'
- blockclass = 'source'
Sink block
- class bdsim.SinkBlock(*args, bd=None, **kwargs)[source]
Bases:
Block
A SinkBlock is a subclass of Block that represents a block that has inputs but no outputs. Typically used to save data to a variable, file or graphics.
- __annotations__ = {}
- __init__(**blockargs)[source]
Create a sink block.
- Parameters:
blockargs (dict) – common Block options
- Returns:
sink block base class
- Return type:
This is the parent class of all sink blocks.
- __module__ = 'bdsim.components'
- blockclass = 'sink'
Function block
- class bdsim.FunctionBlock(*args, bd=None, **kwargs)[source]
Bases:
Block
A FunctionBlock is a subclass of Block that represents a block that has inputs and outputs but no state variables. Typically used to describe operations such as gain, summation or various mappings.
- __annotations__ = {}
- __init__(**blockargs)[source]
Create a function block.
- Parameters:
blockargs (dict) – common Block options
- Returns:
function block base class
- Return type:
This is the parent class of all function blocks.
- __module__ = 'bdsim.components'
- blockclass = 'function'
Transfer function block
- class bdsim.TransferBlock(*args, bd=None, **kwargs)[source]
Bases:
Block
A TransferBlock is a subclass of Block that represents a block with inputs outputs and states. Typically used to describe a continuous time dynamic system, either linear or nonlinear.
- __annotations__ = {}
- __init__(nstates=1, **blockargs)[source]
Create a transfer function block.
- Parameters:
blockargs (dict) – common Block options
- Returns:
transfer function block base class
- Return type:
This is the parent class of all transfer function blocks.
- __module__ = 'bdsim.components'
- blockclass = 'transfer'
Subsystem block
- class bdsim.SubsystemBlock(*args, bd=None, **kwargs)[source]
Bases:
Block
A SubSystem s a subclass of Block that represents a block that has inputs and outputs but no state variables. Typically used to describe operations such as gain, summation or various mappings.
- __annotations__ = {}
- __init__(**blockargs)[source]
Create a subsystem block.
- Parameters:
blockargs (dict) – common Block options
- Returns:
subsystem block base class
- Return type:
This is the parent class of all subsystem blocks.
- __module__ = 'bdsim.components'
- blockclass = 'subsystem'
Graphics block
- class bdsim.GraphicsBlock(*args, bd=None, **kwargs)[source]
Bases:
SinkBlock
A GraphicsBlock is a subclass of SinkBlock that represents a block that has inputs but no outputs and creates/updates a graphical display.
- __annotations__ = {}
- __init__(movie=None, **blockargs)[source]
Create a graphical display block.
- Parameters:
movie (str, optional) – Save animation in this file in MP4 format, defaults to None
blockargs (dict) – common Block options
- Returns:
transfer function block base class
- Return type:
This is the parent class of all graphic display blocks.
- __module__ = 'bdsim.graphics'
- blockclass = 'graphics'
Discrete-time systems
- class bdsim.ClockedBlock(*args, bd=None, **kwargs)[source]
Bases:
Block
A ClockedBlock is a subclass of Block that represents a block with inputs outputs and discrete states. Typically used to describe a discrete time dynamic system, either linear or nonlinear.
- __init__(clock=None, **blockargs)[source]
Create a clocked block.
- Parameters:
blockargs (dict) – common Block options
- Returns:
clocked block base class
- Return type:
This is the parent class of all clocked blocks.
- blockclass = 'clocked'