Mobile robot animations
These classes create a graphical object that can be animated to show vehicle position or pose.
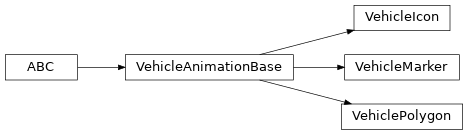
- class roboticstoolbox.mobile.VehicleAnimationBase[source]
Abstract base class to support animation of a vehicle on Matplotlib plot
There are three concrete subclasses:
VehicleMarker
animates a Matplotlib markerVehiclePolygon
animates a polygon shape (outline or filled), including predefined shapesVehicleIcon
animates an image
An instance
a
of these classes can be used in three different ways, firstly:a = VehiclePolygon("car", color="red") a.add()
adds an instance of the animation shape to the plot and subsequent calls to:
a.update(q)
will animate it.
Secondly, an instance can be passed to a Vehicle subclass object to make an animation during simulation:
a = VehiclePolygon("car", color="red") veh = Bicycle(animation=a)
Thirdly:
a = VehiclePolygon("car", color="red") a.plot(q)
adds an instance of the animation shape to the plot with the specified configuration. It cannot be moved, but the method does return a reference to the Matplotlib object added to the plot.
Simple marker
- class roboticstoolbox.mobile.VehicleMarker(**kwargs)[source]
Bases:
VehicleAnimationBase
- __init__(**kwargs)[source]
Create graphical animation of vehicle as a Matplotlib marker
- Parameters
kwargs – additional arguments passed to Matplotlib
plot()
.- Returns
animation object
- Return type
VehicleAnimation
Creates an object that can be passed to a
Vehicle
subclass to depict the moving robot as a simple Matplotlib marker during simulation.The default marker is a red filled circle with a white outline.
For example, to animate a simulation with a blue square marker:
a = VehicleMarker(marker="s", markerfacecolor="b") veh = Bicycle(driver=RandomPath(10), animation=a) veh.run()
Note
A marker can only indicate vehicle position, not orientation.
- Seealso
- add(ax=None, **kwargs)
Add vehicle animation to the current plot
- Parameters
ax (Axes, optional) – Axis to add to, defaults to current axis
kwargs – additional arguments passed to Matplotlib
plot()
, which override arguments given to the constructor.
A reference to the animation object is kept, and it will be deleted from the plot when the
VehicleAnimation
object is garbage collected.The animation is not displayed until
update()
is called.- Seealso
- plot(q, **kwargs)
Add vehicle to the current plot (superclass)
- Parameters
q (array_like(2) or array_like(3)) – vehicle position or configuration
kwargs – additional arguments passed to Matplotlib
plot()
, which override arguments given to the constructor.
- Returns
reference to Matplotlib object
The animation object is rendered into the current axes.
Polygon shape
- class roboticstoolbox.mobile.VehiclePolygon(shape='car', scale=1, **kwargs)[source]
Bases:
VehicleAnimationBase
- __init__(shape='car', scale=1, **kwargs)[source]
Create graphical animation of vehicle as a polygon
- Parameters
shape (ndarray(2,n) or str) – polygon shape as vertices or a predefined shape, defaults to “car”
scale (float) – Length of the vehicle on the plot, defaults to 1
kwargs – additional arguments passed to Matplotlib
Polygon
such ascolor
(face+edge),alpha
,facecolor
,edgecolor
,linewidth
etc.
- Raises
ValueError – unknown shape name
TypeError – bad shape argument
- Returns
animation object
- Return type
Creates an object that can be passed to a
Vehicle
subclass to depict the moving robot as a polygon during simulation.For example, to animate a simulation with a red filled car-shaped polygon:
a = VehiclePolygon("car", color="r") veh = Bicycle(driver=RandomPath(10), animation=a) veh.run()
shape
can be:"car"
a rectangle with chamfered front corners"triangle"
an isocles triangle pointing in the forward directionan 2xN NumPy array of vertices, does not have to be closed.
The polygon is scaled to an image with a length of
scale
in the vehicle x-direction, in the units of the plot.- Seealso
- add(ax=None, **kwargs)
Add vehicle animation to the current plot
- Parameters
ax (Axes, optional) – Axis to add to, defaults to current axis
kwargs – additional arguments passed to Matplotlib
plot()
, which override arguments given to the constructor.
A reference to the animation object is kept, and it will be deleted from the plot when the
VehicleAnimation
object is garbage collected.The animation is not displayed until
update()
is called.- Seealso
- plot(q, **kwargs)
Add vehicle to the current plot (superclass)
- Parameters
q (array_like(2) or array_like(3)) – vehicle position or configuration
kwargs – additional arguments passed to Matplotlib
plot()
, which override arguments given to the constructor.
- Returns
reference to Matplotlib object
The animation object is rendered into the current axes.
Image icon
- class roboticstoolbox.mobile.VehicleIcon(filename, origin=None, scale=1, rotation=0)[source]
Bases:
VehicleAnimationBase
- __init__(filename, origin=None, scale=1, rotation=0)[source]
Create graphical animation of vehicle as an image icon
- Parameters
filename (str) – Standard icon name or a path to an image
origin (array_like(2)) – Origin of the vehicle coordinate frame, defaults to centre
scale (float) – Length of the vehicle on the plot, defaults to 1
rotation (float) – Vehicle icon heading in degrees, defaults to 0
- Raises
ValueError – Icon file not found
- Returns
animation object
- Return type
VehicleAnimation
Creates an object that can be passed to a
Vehicle
subclass to depict the moving robot as an image icon during simulation. The image is translated and rotated to represent the vehicle configuration.The car is scaled to an image with a horizontal length (width) of
scale
in the units of the plot. By default the image is assumed to contain a car parallel to the x-axis and facing right. If the vehicle is facing upward setrotation
to 90.The vehicle rotates about its
origin
which is expressed in terms of normalized coordinates in the range 0 to 1. By default it is in the middle of the icon image, (0.2, 0.5) moves it toward the back of the vehicle, (0.8, 0.5) moves it toward the front of the vehicle.filename
can be an included image:"greycar"
a grey and white car (top view)"redcar"
a red car (top view)"piano"
a piano (top view)
or the path to an image file, including extension.
The included images are:
For example, to animate a simulation with the red car icon:
a = VehicleIcon("redcar", scale=2) veh = Bicycle(driver=RandomPath(10), animation=a) veh.run(animation=a)
Note
The standard icons are provided in the package
rtb-data
- Seealso
- add(ax=None, **kwargs)
Add vehicle animation to the current plot
- Parameters
ax (Axes, optional) – Axis to add to, defaults to current axis
kwargs – additional arguments passed to Matplotlib
plot()
, which override arguments given to the constructor.
A reference to the animation object is kept, and it will be deleted from the plot when the
VehicleAnimation
object is garbage collected.The animation is not displayed until
update()
is called.- Seealso
- plot(q, **kwargs)
Add vehicle to the current plot (superclass)
- Parameters
q (array_like(2) or array_like(3)) – vehicle position or configuration
kwargs – additional arguments passed to Matplotlib
plot()
, which override arguments given to the constructor.
- Returns
reference to Matplotlib object
The animation object is rendered into the current axes.